You may be wondering how you can get started with machine learning. You should begin by learning Python, a minimalist yet elegant programming language. It is easy to learn and has many built-in libraries to help you build your ML models. How do you start learning Python? It is not as hard as you may think.
How To Get Started With Python
The single best way to start learning Python (or any programming language) is to build projects. You can learn a lot from online tutorials. However, once you start coding yourself, you will understand these concepts in a whole new depth.
Important disclaimer — If you are anything like me, your first projects will be elementary and limited in scope.
This is okay, though. Remember that the goal of these projects is to build skills, nothing else. Thus, you should start small and build up. To get some ideas, be sure to check out the following articles:
- Six Python Projects For Beginners
- Best Python Projects For Beginners in 2020
- Python Project Ideas for 2020
If you are still unsure about what to build, read onward! I will walk you through the step-by-step details of building your first game in Python.
First Python Project: Step by Step Tutorial
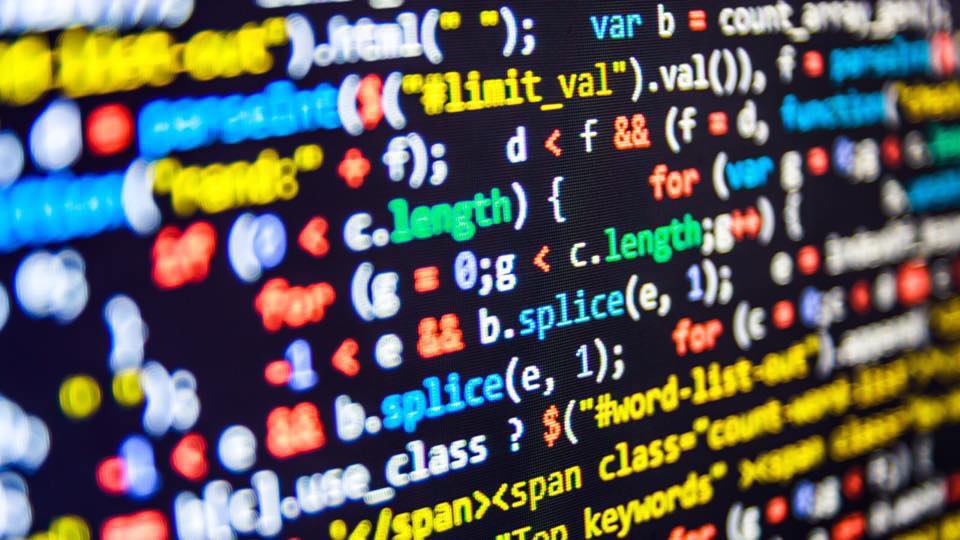
We will be building a simple guessing game in python. The objective of the game will be to guess a randomly generated number within a preset range. Let’s break our game down into steps:
- Randomly generate a number.
- Ask the user to guess the number.
- Compare the user’s guess with the actual number.
- Ask the user to guess again if wrong.
- End the game after too many incorrect guesses.
We will now proceed to implement these steps one by one.
Step 1. Randomly Generate a Number
Import the random module into Python and generate a pseudo-random integer.
import random print("Welcome to Zach's guessing game!!") #DEFINE CONSTANTS MIN_VALUE = 1 MAX_VALUE = 10 #GENERATE RANDOM NUMBER number = random.randint(MIN_VALUE, MAX_VALUE)
Step 2. Prompt User For Guess
Ask the user to guess the number with input().
import random print("Welcome to Zach's guessing game!!") #DEFINE CONSTANTS MIN_VALUE = 1 MAX_VALUE = 10 #GENERATE RANDOM NUMBER number = random.randint(MIN_VALUE, MAX_VALUE) #PROMPT THE USER TO MAKE A GUESS print("I am thinking number between {mini} and {maxi}\nCan you guess it?".format(mini=MIN_VALUE, maxi=MAX_VALUE)) guess = int(input())
Step 3. Compare the Guess to the Actual Number
Use an if/else statement to compare the user’s guess to the real number. Print the appropriate message.
import random print("Welcome to Zach's guessing game!!") #DEFINE CONSTANTS MIN_VALUE = 1 MAX_VALUE = 10 #GENERATE RANDOM NUMBER number = random.randint(MIN_VALUE, MAX_VALUE) #PROMPT THE USER TO MAKE A GUESS print("I am thinking number between {mini} and {maxi}\nCan you guess it?".format(mini=MIN_VALUE, maxi=MAX_VALUE)) guess = int(input()) if guess == number: print("You guessed my number. YOU WIN!!") elif guess > number: print("Your guess is too large") else: print("Your guess is too small")
Step 4. Ask the User to Guess Again if Wrong
Implement a while loop to get the user to guess again if her initial guess is wrong.
import random print("Welcome to Zach's guessing game!!") #DEFINE CONSTANTS MIN_VALUE = 1 MAX_VALUE = 10 MAX_TRIES = 3 number = random.randint(MIN_VALUE, MAX_VALUE) tries = 0 print("I am thinking number between {mini} and {maxi}\nCan you guess it?".format(mini=MIN_VALUE, maxi=MAX_VALUE)) #GAME LOOP while tries < MAX_TRIES: #PROMPT USER FOR A GUESS guess = int(input()) if guess == number: print("You guessed my number. YOU WIN!!") break elif guess > number: print("Your guess is too large") else: print("Your guess is too small") #INCREMENT TRIES tries += 1
Step 5. End the Game After Too Many Incorrect Guesses
If the user fails to guess the correct number after a certain number of tries, we want to end the game. We can do this with a simple if statement after the while loop.
import random print("Welcome to Zach's guessing game!!") #DEFINE CONSTANTS MIN_VALUE = 1 MAX_VALUE = 10 MAX_TRIES = 3 number = random.randint(MIN_VALUE, MAX_VALUE) tries = 0 print("I am thinking number between {mini} and {maxi}\nCan you guess it?".format(mini=MIN_VALUE, maxi=MAX_VALUE)) #GAME LOOP while tries < MAX_TRIES: #PROMPT USER FOR A GUESS guess = int(input()) if guess == number: print("You guessed my number. YOU WIN!!") break elif guess > number: print("Your guess is too large") else: print("Your guess is too small") #INCREMENT TRIES tries += 1 #TOO MANY UNSUCCESSFUL TRIES if (tries >= MAX_TRIES): print("You have used all of your tries. YOU LOSE.")
Testing and Debugging the Code
If you’ve carefully followed all of the steps above, you should be ready to run your code. Upon doing so, you should see the following output:
Now try guessing the number. You should be able to get it after a couple of tries:
Finally, you should check to make sure that the game ends if you make 3 unsuccessful guesses:
You should check to make sure that the above features work on your program.
If your code does not run or a feature is not working for you, don’t despair! Try troubleshooting the issue by looking it up on Stack Overflow or Quora.
If the problem still is not resolved, try adding some print statements to your code to ensure that each part works as intended. As a last resort, you can copy and paste the code from above. However, I strongly advise against this approach as you will learn best by writing the code yourself.
Conclusion and Further Applications
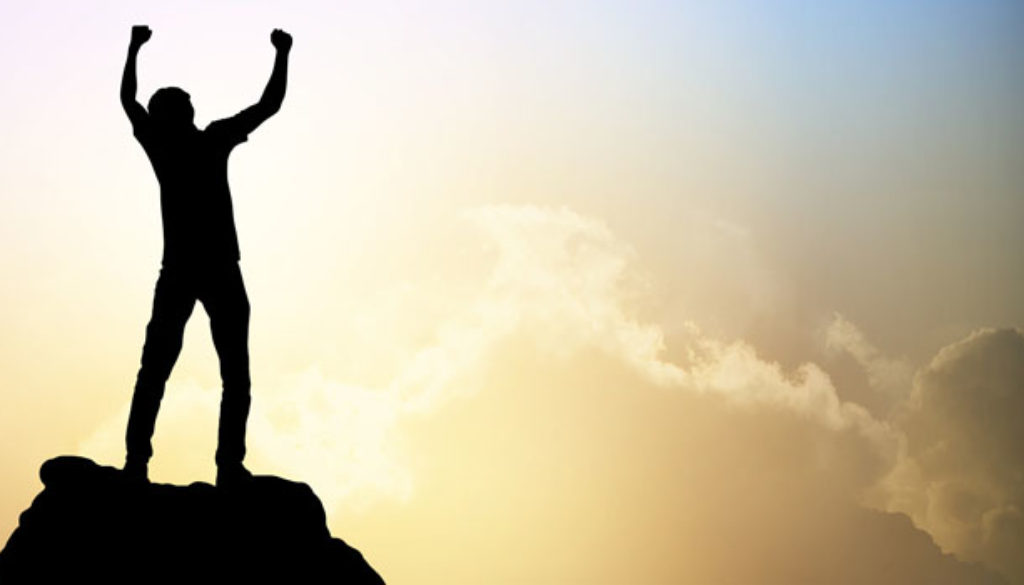
Congratulations on making your first game in Python!!
You should feel proud of yourself. You have solidified your understanding of key topics in Python such as:
- Importing Modules
- Variable declaration
- If/else statements
- While loops
I encourage you to tinker around with the above code. Try adding additional features to enhance the quality of the game. Here are some ideas to get you started:
- Add error messages when the user enters a non-integer value
- Give the user hints to help him guess the number
- Challenge!!! Create an AI bot that guesses the number 🙂
Thank you for reading and be sure to subscribe to our mailing list.